semaphores, shared memory
Files
open()
/
close()
opens and closes a file through its descriptor.
flags:
O_RDONLY
,
O_WRONLY
,
O_RDWR
,
0_CREAT
(create if it doesn’t exist),
0_EXCL
(fail if it exists)
“truncate” in the man-pages means the content of the file gets deleted.
read and write n bytes with
read()
/write()
→
can be interrupted with
EINTR
.
fopen()
/
fclose()
open and close a file stream (operates through a buffer).
empty the buffer and write content with
fflush()
or
fclose()
.
remove the buffer altogether with
setvbuf()
get file descriptor of a stream with
fileno()
.
read and write into buffer with:
fread()
,fgets()
,fgetc()
,fputs()
,fputc()
,fprintf()
,getline()
→
can be interrupted with
EINTR
.
Semaphores
sem_open()
server: create
sem_t *sem = sem_open("/name", O_CREAT | O_EXCL, S_IRUSR | S_IWUSR, <initial value>);client: open
sem_t *sem = sem_open("/name", 0);
sem_wait()
/
sem_post()
blocks process if value is not positive.
processes are not queued up and the order of unblocking is not defined.
can be interrupted with
EINTR
.
sem_unlink()
removes semaphore
Shared memory
/dev/shm/myshm
= shared memory / temporary files that get mapped into virtual memory of multiple processes.
/proc/PID/maps
= mappings.
shm_open()
creates / opens shared memory
ftruncate()
sets size
mmap()
maps the data structures to the process address space
close(shmfd)
uses file descriptor to close
shm_unlink()
frees memory
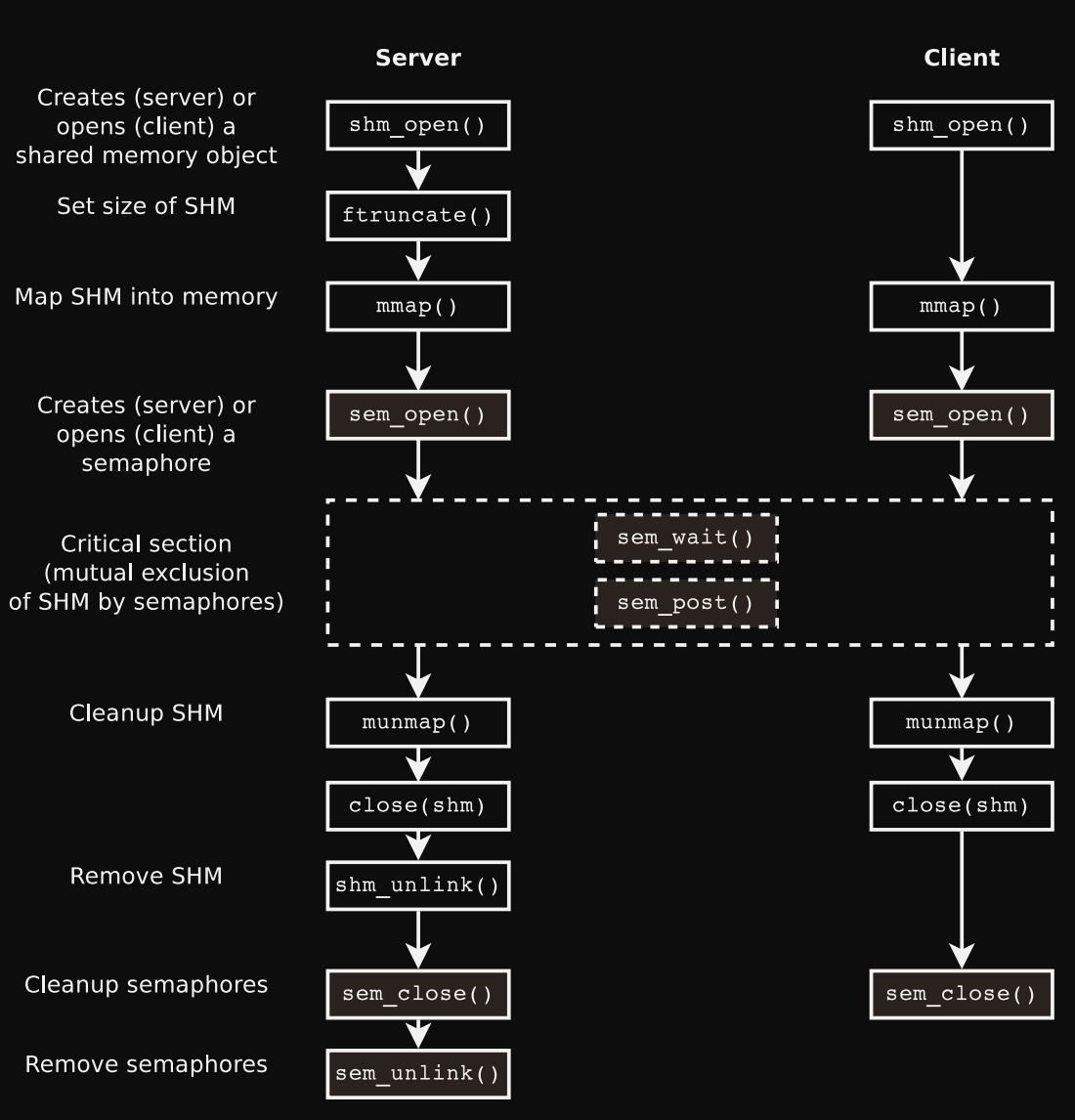
Circular buffer
concurrent data structure using an array where the modulo operator is used.
int wr_pos = 0;void write(int val) {
sem_wait(free_sem);
buf[wr_pos] = val;
sem_post(used_sem);wr_pos += 1;
wr_pos %= sizeof(buf);
}int rd_pos = 0;void read() {
sem_wait(used_sem);
int val = buf[rd_pos];
sem_post(free_sem);rd_pos += 1;
rd_pos %= sizeof(buf);
}