OpenMP in C
Compiling
Header
<omp.h>
Compiling and setting environment variables
Most C compilers now support OpenMP, usually no special support needed.
gcc -Wall -fopenmp -o openmphello –O3 openmphello.c
Setting environment variables
Setting default number of threads
(Listed in increasing priority)
-
Environment variable in shell
can be changed by environment variable
OMP_NUM_THREADS
intcshell/scshell/bash
#!/bin/bash setenv OMP_NUM_THREADS t
-
Explicit setting by library call
omp_set_num_threads(t);
-
Clause
num_threads(t)
in#pragma omp parallel
Accessing thread number
Library routines for explicit thread number access
Master thread always has number 0.
#include <omp.h>void omp_set_num_threads(int num_threads);
int omp_get_num_threads(void);
int omp_get_max_threads(void);
int omp_get_thread_num(void);
int omp_get_num_procs(void);
Example: Set number of threads and print thread ID
#include <stdio.h> #include <stdlib.h> #include <omp.h> // OpenMP headerint main(int argc, char *argv[]) { int threads, myid; int i; threads = 1; // shared variable // read from commandline for (i=1; i<argc && argv[i][0]=='-'; i++) { if (argv[i][1]=='t') { sscanf(argv[++i],"%d",&threads); } }printf("Maximum number of threads possible is %d\n", omp_get_max_threads());// check if below max, else set to max if (threads < omp_get_max_threads()) { if (threads<1) { threads = 1; } omp_set_num_threads(threads); } else { threads = omp_get_max_threads(); } #pragma omp parallel num_threads(threads) { int myid = omp_get_thread_num(); // private variable printf("Thread %d of %d active\n", myid, threads); }return 0; }
Benchmarking
Timing computations for benchmarking
used for performance evaluation:
- thread safe
- non deterministic: influenced by OS and other active users.
Wall-clock time in seconds since some time in the past returned by
omp_get_wtime()
.
#include <omp.h>double omp_get_wtime(void);
double omp_get_wtick(void);
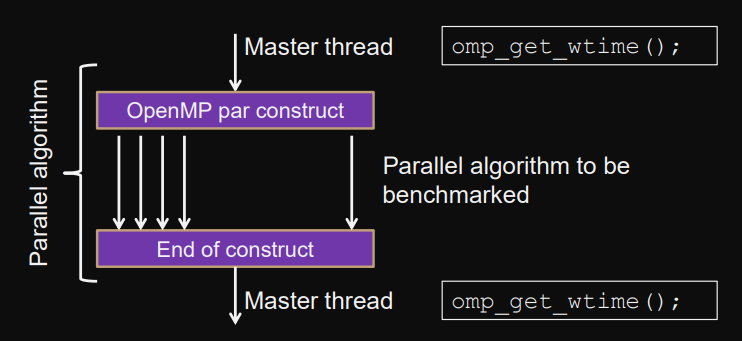