DOM
Definition
DOM
= Document Object Model
Tree-based parser
converts document into XML DOM-object that can be accessed via the API.
W3C standard for reading, changing, adding, deleting XML elements.
Tree based Parser
Map XML document to internal tree structure in main memory.
App can read structure from main memory.
Used for small documents with ready-made data structure
- Random access
- Slow
- Occupied memory constant to document size
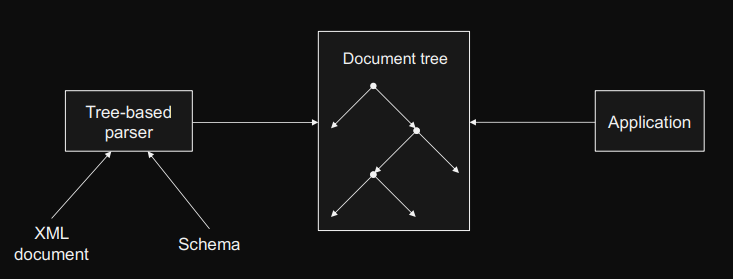
Node Interface
Loading an XMLDocument
import javax.xml.parsers.*;
import org.w3c.dom. *;
public class Course {public static void main(String[] args) throws Exception {
//factory instantiation: API that enables applications to obtain a parser that
//produces DOM object trees from XML documents
DocumentBuilderFactory factory = DocumentBuilderFactory.newInstance();
//validation and namespaces
factory.setValidating(true);
factory.setNamespaceAware(true);
//parser instantiation
//API to obtain DOM document instances from XML documents
DocumentBuilder builder = factory.newDocumentBuilder();
//install ErrorHandler
builder.setErrorHandler(new MyErrorHandler());
//parsing instantiation (code below is written from this line onwards)
Document coursedoc = builder.parse(args[0]);
}
}
The
Node
interface is the datatype of the entire DOM.
More specific subinterfaces inherit from it:
Document
,
Element
,
Attr
,
Text
, ...
Example
Visit all child nodes of “courses.xml”
visitNode(coursedoc.getDocumentElement());private void visitNode(Node node) { //iterate over all children for (int i = 0; i < node.getChildNodes().getLength(); i++) { //recursively visit all nodes visitNode(node.getChildNodes().item(i)); } }
Node Interface
Document Methods (child interface of Node)
Element Methods (child interface of Node)
Attr Methods (child interface of Node)
Example
<?xml version="1.0"?>
<courses>
<course semester="Summer">
<title> Semi-structured Data (SSD) </title>
<day> Thursday </day>
<time> 09:15 </time>
<location> HS8 </location>
</course>
</courses>
courses:
course: semester="Summer"
title: "Semi-structured Data (SSD)"
day: "Thursday"
time: "09:15"
location: "HS8"
Reading document
import jave.io.*; import javax.xml.parsers.*; import org.w3c.dom. *;public class Course {public static void main(String[] args) throws Exception { // ... see above ...Document coursedoc = builder.parse(args[0]);//call visit node starting from the root node visitNode(coursedoc.getDocumentElement()); }//the recursive method visitNode private static void visitNode(Node node) { //element nodes if (node.getNodeType() == Node.ELEMENT_NODE) { System.out.print("\n" + node.getNodeName() + ": "); NamedNodeMap attributes = node.getAttributes(); if (attributes != null) { for (int i = 0; i < attributes.getLength(); i++) { System.out.print(attributes.item(i) + " "); } } } //text nodes if (node.getNodeType() == Node.TEXT_NODE && !node.getTextContent().trim().isEmpty()) { System.out.print("\"" + node.getTextContent().trim() + "\""); } // visit child nodes NodeList nodelist = node.getChildNodes(); for (int i = 0; i < nodelist.getLength(); i++) { visitNode(nodelist.item(i)); } } }
Create and validate document
import java.io.File; import javax.xml.parsers.*; import javax.xml.transform.*; import javax.xml.transform.dom.DOMSource; import javax.xml.transform.stream.StreamResult; import org.w3c.dom.*; public class Course {public static void main(String[] args) throws Exception { // ... see above .../* * CREATE DOCUMENT */ Document coursedoc = builder.newDocument();//create all the necessary elements Element courses = coursedoc.createElement("courses"); Element course = coursedoc.createElement("course"); course.setAttribute("semester", "Summer"); Element title = coursedoc.createElement("title"); title.setTextContent("Semi-structured Data (SSD)");//append the children in a bottom-up-order course.appendChild(title); course.appendChild(day); course.appendChild(time); course.appendChild(location); courses.appendChild(course); coursedoc.appendChild(courses);/* * VALIDATE */ //validate the new document against a schema //load XML Schema File schemaFile = new File("schema.xsd"); SchemaFactory factory= SchemaFactory(XMLConstants.W3C_XML_SCHEMA_NS_URI); Schema schema = schemaFactory.newSchema(schemaFile); // Create Validator and validate Validator validator = schema.newValidator(); try{ validator.validate(new DOMSource(coursedoc)); } catch (SAXException ex) { exit("Created document not valid:" + ex.getMessage()); }/* * WRITE INTO A FILE */ //write the document into a file //factory instantiation TransformerFactory tfactory = TransformerFactory.newInstance(); //transformer instantiation Transformer transformer = tfactory.newTransformer(); //create a new input XML source DOMSource source = new DOMSource(coursedoc); //construct a stream result StreamResult result = new StreamResult(new File("courses.xml")); //actual transformation transformer.transform(source, result); System.out.println("File saved!"); } }