XSLT
Definition
XSLT
XSL = eXtensible Stylesheet Language for XML (like CSS for HTML)
XSLT = XSL Transformations / templates
XML documents that match the input document and define the output:
XML documentXML/HTML/txt document
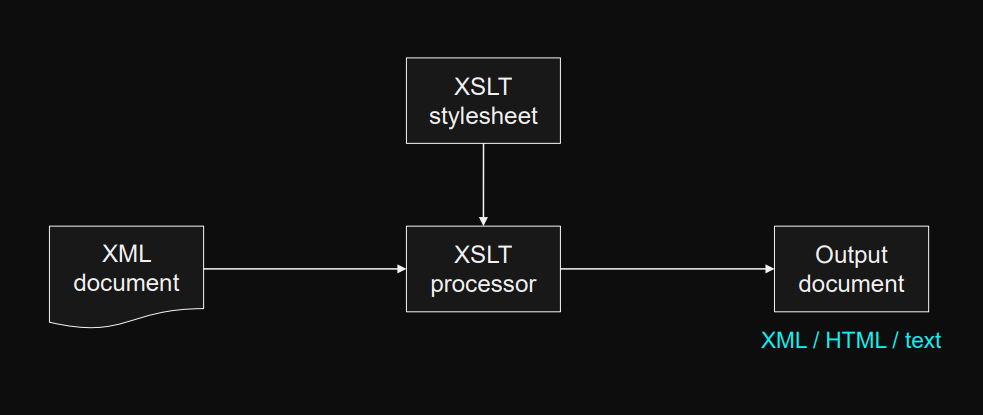
Example
<courses> <course semester="Summer"> <title> SSD </title> <day> Thursday </day> <time> 09:15 </time> <location> HS8 </location> </course> <course semester="Winter"> <title> Databases </title> <day> Tuesday </day> <time> 09:15 </time> <location> HS8 </location> </course> </courses>
<html> <head> <title>Lectures Overview</title> </head> <body> <h1>DBAI Lectures</h1> <table> <tr><th>Semester</th><th>Title</th><th>Date / Time</th><th>Location</th></tr> <tr><td>Summer</td><td>SSD</td><td>Thursday, 09:15</td><td>HS8</td></tr> <tr><td>Winter</td><td>Databases</td><td>Thursday, 09:15</td><td>HS8</td></tr> </table> </body> </html>
Through the XSLT document that matches nodes:
<?xml version="1.0"?> <xsl:stylesheet version="1.0" xmlns:xsl=“http://www.w3.org/1999/XSL/Transform”> <xsl:output version="html"/><xsl:template match="courses"> <html> <head> <title>Lectures Overview</title> </head> <body> <h1>DBAI Lectures</h1> <table> <tr><th>Semester</th><th>Title</th><th>Date / Time</th><th>Location</th></tr> <xsl:apply-templates select="course"/> </table> </body> </html> </xsl:template> <xsl:template match="course"> <tr> <td><xsl:value-of select="@semester"/></td> <td><xsl:value-of select="title"/></td> <td><xsl:value-of select="day"/>, <xsl:value-of select="time"/></td> <td><xsl:value-of select="location"/></td> </tr> </xsl:template></xsl:stylesheet>
XSLT Templates
Template
<xsl:
template
match="
pattern
">
match element nodes, replace with content
does not apply pattern to children of the node - must be done explicitly:
<xsl:
apply-templates
select="
xPath expression
">
Default template
When template matches element without any instructions, then the default template (which is always present) gets applied:
elements apply childrens template to parents
text copy content to the output
attributes copy value to the output
Priorisation of templates
Only 1 template is applied based on its priority value (defined by the specificity of XPath expression)
If ambiguious (two templates get matched for the same element) then this causes an error.
Named templates
<xsl:
call-template
name="...">
used to avoid writing the same template for multiple elements
<xsl:template match="name | email">
<xsl:call-template name="output"/>
</xsl:template><xsl:template name="output">
Below you'll see either a name or mail:
<xsl:value-of select="."/>
</xsl:template>
Creating output
<xsl:element name="...">
create an element
<xsl:attribute name="...">
create an attribute
<xsl:text name="...">
create a text
<xsl:comment name="...">
add a comment
Example using all
<courses> <course> <title> SSD </title> <day> Thursday </day> <time> 09:15 </time> </course> <course> <title> Databases </title> <day> Tuesday </day> <time> 09:15 </time> </course> </courses>
<courses> <!-- Course Description --> <SSD day="Thu"> Starts at 09:15 </SSD> <Databases day="Tue"> Starts at 09:15 </Databases> </courses>
Template:
<xsl:template match="courses"> <courses> <xsl:comment> Course Description </xsl:comment> <xsl:apply-templates select="course"/> </courses> </xsl:template><xsl:template match="course"><xsl:element name="{title/text()}"> <xsl:attribute name="day"> <xsl:value-of select="day"/> </xsl:attribute><xsl:text> Starts at <xsl:value-of select="time"/> </xsl:text></xsl:element></xsl:template>
Other Features
<xsl:for-each select="...">
select and iterate
<xsl:copy-of select="...">
creates a deep-copy of the selected node
<xsl:sort select=".." order="..">
sorts ascending / descending (used in
for-each
loop)
<xsl:if test="...">
applies content if
test
is evaluated to true
<xsl:choose select="...">
uses
<xsl:when test="...">
and
<xsl:otherwise>
<xsl:variable select="...">
used to bypass restrictions with complex XPath expressions